Home » Renegade Discussions » Mod Forum » Working vehicle menus on SP maps, and how to purchase powerups from them and airdrop vehicles
( ) 1 Vote
Working vehicle menus on SP maps, and how to purchase powerups from them and airdrop vehicles [message #467296] |
Sat, 12 May 2012 02:46  |
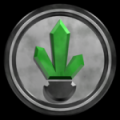 |
Whitedragon
Messages: 832 Registered: February 2003 Location: California
Karma: 1
|
Colonel |
|
|
I've noticed that the way vehicles and powerups are bought on coop servers is kinda ugly, using pokeable objects and chat commands. So here's some code that will allow you to have working vehicle purchase menus on SP maps. You will be able to purchase vehicles from the PT and have them airdropped in, and purchase powerups the same way as beacons. It could also be made to airdrop the powerups if you wanted to.
void Set_Vehicle_Factory_Is_Busy(VehicleFactoryGameObj *VF,bool Busy) {
if (VF) {
*((bool*)VF+0x8C8) = Busy; //Set_Is_Busy needs to be added to VehicleFactoryGameObj
VF->Set_Object_Dirty_Bit(NetworkObjectClass::BIT_RARE,true);
}
}
int VehiclePurchase(BaseControllerClass *Base,GameObject *Purchaser,unsigned int Cost,unsigned int Preset,const char *Data) {
DefinitionClass *Def = Find_Definition(Preset);
if (Def) {
if (Def->Get_Class_ID() == CID_Vehicle) { //It's a vehicle
VehicleFactoryGameObj *VF = (VehicleFactoryGameObj*)Base->Find_Building(BuildingConstants::TYPE_VEHICLE_FACTORY);
if (!Base->Can_Generate_Vehicles() || !VF || VF->Is_Busy()) {
return 3;
}
else if ((unsigned int)VF->Get_Team_Vehicle_Count() >= Get_Vehicle_Limit()) {
return 3;
}
if (Purchase_Item(Purchaser,Cost)) {
int Team = Base->Get_Player_Type();
VehicleGameObj *Veh = Commands->Create_Object(Def->Get_Name(),Vector3(0,0,0))->As_VehicleGameObj();
GameObject *Cin = Commands->Create_Object("Invisible_Object",VehiclePosition[Team]); //Set the position however you want
Commands->Attach_Script(Cin,"Test_Cinematic",StringClass::getFormattedString("%s_Vehicle_Purchase.txt",Team?"GDI":"Nod")); //Create the cinematic
Commands->Send_Custom_Event(Veh,Cin,10004,Commands->Get_ID(Veh),0); //Insert vehicle into cinematic at slot 4
Veh->Lock_Vehicle(Purchaser->As_SoldierGameObj(),30.0f); //Lock vehicle
Set_Vehicle_Factory_Is_Busy(VF,true);
//You should attach a script to the vehicle that makes it invincible until it lands. The same script can also be used to make the VF not busy.
return 0;
}
return 2;
}
else if (Def->Get_Class_ID() == CID_PowerUp) { //It's a powerup
PowerUpGameObjDef *PowerUpDef = (PowerUpGameObjDef*)Def;
if (PowerUpDef->GrantWeapon && Has_Weapon(Purchaser,Get_Definition_Name(PowerUpDef->GrantWeaponID))) {
return 4; //Stop players from accidentally buying a weapon they already have
}
else if (Purchase_Item(Purchaser,Cost)) {
PowerUpDef->Grant(Purchaser->As_SoldierGameObj());
return 0;
}
return 2;
}
}
return 4;
}
Obviously you'll need a vehicle factory on the map which can be added through leveledit or created in a script with Create_Building.
Black-Cell.net
Network Administrator (2003 - )
DragonServ, Renegade's first IRC interface bot
Creator and lead coder (2002 - )
Dragonade, Renegade's first server side modification
Lead coder (2005 - )
[Updated on: Tue, 15 May 2012 02:54] Report message to a moderator
|
|
|
|
|
|
Re: Working vehicle menus on SP maps, and how to purchase powerups from them and airdrop vehicles [message #467357 is a reply to message #467296] |
Mon, 14 May 2012 13:26   |
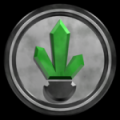 |
Whitedragon
Messages: 832 Registered: February 2003 Location: California
Karma: 1
|
Colonel |
|
|
Oh yeah, here's the character purchase hook so you can have powerups in the character menu too.
int CharacterPurchase(BaseControllerClass *Base,GameObject *Purchaser,unsigned int Cost,unsigned int Preset,const char *Data) {
if (Base->Can_Generate_Soldiers() || !Cost) {
DefinitionClass *Def = Find_Definition(Preset);
if (Def->Get_Class_ID() == CID_Soldier) {
if (Purchase_Item(Purchaser,Cost)) {
Purchaser->As_SoldierGameObj()->Re_Init(*(SoldierGameObjDef*)Def);
Purchaser->As_SoldierGameObj()->Post_Re_Init();
return 0;
}
return 2;
}
else if (Def->Get_Class_ID() == CID_PowerUp) {
PowerUpGameObjDef *PowerUpDef = (PowerUpGameObjDef*)Def;
if (PowerUpDef->GrantWeapon && Has_Weapon(Purchaser,Get_Definition_Name(PowerUpDef->GrantWeaponID))) {
return 4;
}
else if (Purchase_Item(Purchaser,Cost)) {
PowerUpDef->Grant(Purchaser->As_SoldierGameObj());
return 0;
}
return 2;
}
}
return 3;
}
Black-Cell.net
Network Administrator (2003 - )
DragonServ, Renegade's first IRC interface bot
Creator and lead coder (2002 - )
Dragonade, Renegade's first server side modification
Lead coder (2005 - )
|
|
|
|
Goto Forum:
Current Time: Thu Jul 17 20:20:39 MST 2025
Total time taken to generate the page: 0.00706 seconds
|