distance between two objects c++ [message #432316] |
Thu, 08 July 2010 04:37  |
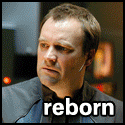 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
Someone asked me about setting a conditional that checked whether the distance between two objects was lower than 40 metres. They aaked me privately and for it in LUA, but I prefer this in the public domain, there could be others that have the same question or maybe someone else might have something useful to add...
Besides, they also said they would of posted the question in another forum that is public, so go figure? Fucking leachers!
I do not know how to write this in LUA, but here it is in C++:
//obj1 and obj2 are the Two GameObject's you're comparing.
float Dist;
Vector3 pos1, pos2;
pos1 = Commands->Get_Position(obj1);
pos2 = Commands->Get_Position(obj2);
Dist = Commands->Get_Distance(pos1, pos2);
if(Dist <= 40.0f){ // If the distance is less than 40 metres
// do something
}
else{ // Distance is greater than 40 metres
// do nothing?
}
or:
float Dist = Commands->Get_Distance(Commands->Get_Position(obj1),Commands->Get_Position(obj2));
if(Dist <= 40.0f){ // If the distance is less than 40 metres
// do something
}
else{ // Distance is greater than 40 metres
// do nothing?
}
or:
if((Commands->Get_Distance(Commands->Get_Position(obj1),Commands->Get_Position(obj2))) <= 40.0f){ // If the distance is less than 40 metres
// do something
}
All of the above should work (not tested), but each show you basically the same thing using less lines.
[Updated on: Fri, 09 July 2010 13:09] Report message to a moderator
|
|
|
Re: distance between two objects c++ [message #432329 is a reply to message #432316] |
Thu, 08 July 2010 07:41   |
Ephphatha
Messages: 1 Registered: June 2010
Karma: 0
|
Recruit |
|
|
For those interested in the math, the distance between two points is the magnitude of the vector from point A to point B.
Taking the first example, the distance between obj1 and obj2 would be calculated:
Vector3 pos1, pos2;
pos1 = Commands->Get_Position(obj1);
pos2 = Commands->Get_Position(obj2);
Vector3 gap = pos1 - pos2; //Doesn't matter which order the subtraction is in.
float dist = gap.length(); //Where length() returns the magnitude of the vector (is this in the sdk?)
And the magnitude is calculated by taking the square root of the sum of the square of each element.
class Vector3
{
public:
float x, y, z;
float length()
{
return sqrt(x*x + y*y + z*z);
}
}
So if there is no length() or equivalent function, you can still get the distance if you have access to each element of the vector.
|
|
|
|
|
|
|
|
|
Re: distance between two objects c++ [message #432346 is a reply to message #432343] |
Thu, 08 July 2010 10:00   |
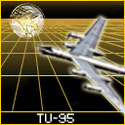 |
Tupolev TU-95 Bear
Messages: 1176 Registered: April 2009 Location: Rìoghachd Aonaichte
Karma: 1
|
General (1 Star) |
|
|
KaTaNa wrote on Thu, 08 July 2010 17:56 | Can anyone offer some real support and stay on topic?
|
Actually, reborn has been nothing but a big help in the forums and this is his thanks?
The only support is reborn.
Decent people = Reaver11, ErroR, all of the mods and admins, DRNG Naffets199, Amber, Dover, GEORGE ZIMMER,Zeratul, Starbuzzzz, kane,R315r4z0r,gnoepower, Danpaul88,HaTe,WNxVerty Tiberian Technologies Generalcamo,  ,  . If you want me to add you on the decent peoples list PM me.
Quotes or w/e Toggle
Zeratul2400 | .AIRCRAFT KILLER YOU AR NOT JUST A BIG JACKAS AND YOU THE BIG HEAD JUST YOU CREATE GLACIER FLAYING FUCK YOU BIG JAKAS YOUR MAPS IS BAD YOU WANT I WRUGHT THIS THE MAPS IS BAD HEY IS 1 YEAR
YOUR PROMESS A CON YARD WIRH A SINGLE PLAYER POWER PLANT IN ONE
MAPS AND IS DONT JUST ACK I TELL IT ALL REPLYER IN THIS FORUM I DESLIKE YOUâ„¢ 2003
|
Zeratul PT2 zeratul2400 wrote on Mon, 10 November 2003 09:49 | GENOCIDE YOU AR NOT JUST A BIG JACKAS AND YOU THE BIG HEAD JUST YOU CREATE DUSK FLAYING FUCK YOU BIG JAKAS YOUR MAPS IS BAD YOU WANT I WRUGHT THIS THE MAPS IS BAD HEY IS ONE YEAR YOUR PROMESS A WARPATH MOD WERKING BUT IT NO WORK AND DONT JUST GENOCIDE I TELL IT ALL REPLYER IN THIS FORUM I DESLIKE YOU.
|
lolnikki6ixx wrote on Wed, 09 June 2010 05:11 | With only 14 people living in Canada, the retarded one was bound to show up around here sooner or later.
|
HaTe wrote on Sun, 04 July 2010 16:17 |
Good-One-Driver wrote on Sun, 04 July 2025 01:37 | everything is possible just need to know how to code
|
Jumping from the empire state building onto concrete head first and surviving is possible if I know how to code? Wow, teach me please.
|
CarrierII wrote on Mon, 19 November 2007 22:35 | I am in a bad mood with you, you wasted my time.
|
Altzan wrote on Wed, 30 September 2009 14:10 |
Dreganius wrote on Wed, 30 September 2009 07:27 |
Good-One-Driver wrote on Wed, 30 September 2009 09:03 | lol fuckin russians damn communists
|
That was the worst comment to this thread ever.
|
Nightma12 wrote on Wed, 08 June 2011 14:27 | your mum is a stupid language
|
|
reborn wrote on Sun, 20 November 2011 11:54 | Liquedv2, yew need to branch out a little on your thinking. I'm sure you'll twig it soon enough, but you're barking up the wrong tree.
The map is budding with new ideas and great game-play, AitcraftKiller just has to get to the root of the problem, it seems to have him pretty stumped right now.
Leaf him alone, he's doing a good job. I'm going to go out on a limb here, and say this map isn't fir you.
Anyway, this thread is really starting to lumber along, all this over some sap's map? I thought the game was dead, but this thread at least is poplar. It makes me pine for the days of yonder.
At the end of the day, life's a Beech.. Who wood of thought it?
|
Ethenal wrote on Sun, 25 March 2012 16:53 |
Azazel wrote on Sun, 25 March 2012 10:20 | 6 Players don't know how to use a tank.
|
But 1 knows how to use triggerbot!
|
|
|
|
|
|
|
|
|
Re: distance between two objects c++ [message #432408 is a reply to message #432316] |
Fri, 09 July 2010 02:01   |
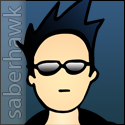 |
saberhawk
Messages: 1068 Registered: January 2006 Location: ::1
Karma: 0
|
General (1 Star) |
|
|
Also, a rather useful optimization when you don't need the exact length (like if you are comparing distances) is to use the squared length instead of the actual length. By doing so you can avoid the square root operation which is rather expensive.
|
|
|
Re: distance between two objects c++ [message #432409 is a reply to message #432316] |
Fri, 09 July 2010 02:16   |
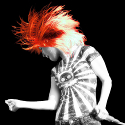 |
danpaul88
Messages: 5795 Registered: June 2004 Location: England
Karma: 0
|
General (5 Stars) |
|
|
Here's a novel idea, why not explain the context of your request. Are you trying to get the distance in a script, in which case you can use the GameObject pointers passed to the script as parameters, or are you using some sort of hooks or what?
Also, if your getting the distance between two game objects quite a lot in your code it might be worth adding a function to do it to improve your code readability. Personally I never understood why something like this was NOT in scripts.dll....
float Get_Distance ( GameObject* obj1, GameObject* obj2 )
{
// Gets distance between two objects
return Commands->Get_Distance ( Commands->Get_Position ( obj1 ), Commands->Get_Position ( obj2 ) );
}
Might also be useful to have
float Get_Distance ( GameObject* obj, Vector3 pos )
{
// Gets distance object and position
return Commands->Get_Distance ( Commands->Get_Position ( obj ), pos );
}
To get the distance between a GameObject and an arbitrary position.
[Updated on: Fri, 09 July 2010 02:19] Report message to a moderator
|
|
|
Re: distance between two objects c++ [message #432437 is a reply to message #432316] |
Fri, 09 July 2010 12:29   |
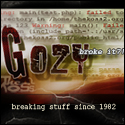 |
Goztow
Messages: 9754 Registered: March 2005 Location: Belgium
Karma: 14
|
General (5 Stars) Goztoe |
|
|
Reborn, may I suggest you take out the private stuff out of your posts? I Understood the person who sent them to you prefers them to stay private and they aren't really needed for the code?
You can find me in The KOSs2 (TK2) discord while I'm playing. Feel free to come and say hi! TK2 discord
|
|
|
|
|