Home » Renegade Discussions » Mod Forum » ion storm effect function
ion storm effect function [message #300900] |
Tue, 04 December 2007 04:06  |
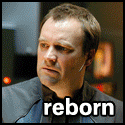 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
I created a function that looks like an ion storm to clients.
To fully appreciate it the client needs to have bhs.dll, as I make use of the fog. But it still looks pretty cool without the fog to be honest.
I was going to put this into an AOW mod I was working on, I still might in time, but I really need to take care of some things first.
Here's a link to a movie of it (don't worry about the end credits and such, I couldn't be bothered to re-make the movie).
http://www.mp-gaming.com/reborn/movies/ion_storm.wmv
Here is how I coded the function:
//GDI version
void reb_GDI_ion_storm::Created(GameObject *obj) {
// And he said "let there be weather!"
Commands->Set_Rain(10.0f,3.5f,true);
Commands->Set_Fog_Enable(1);
Commands->Set_Fog_Range (0.5,55,3.5f);
Commands->Set_Wind(0.7f,2.0f,1.0f,3.5f);
char ionstormmsg[128];
char ionstormmsg2[128];
Commands->Create_2D_WAV_Sound("m00evag_dsgn0069i1evag_snd.wav");
sprintf(ionstormmsg,"msg Warning!!!");
Console_Input(ionstormmsg);
sprintf(ionstormmsg2,"msg Ion Storm approaching...");
Console_Input(ionstormmsg2);
Commands->Start_Timer(obj,this,3.0f,1);
Commands->Start_Timer(obj,this,4.0f,2);
Commands->Start_Timer(obj,this,4.7f,3);
Commands->Start_Timer(obj,this,14.7f,4);
Commands->Start_Timer(obj,this,15.7f,5);
Commands->Start_Timer(obj,this,16.7f,6);
Commands->Start_Timer(obj,this,17.7f,7);
Commands->Start_Timer(obj,this,18.7f,8);
Commands->Start_Timer(obj,this,19.7f,9);
Commands->Start_Timer(obj,this,20.4f,10);
Commands->Start_Timer(obj,this,21.4f,11);
Commands->Start_Timer(obj,this,22.0f,12);
Commands->Start_Timer(obj,this,23.0f,13);
Commands->Start_Timer(obj,this,23.6f,14);
Commands->Start_Timer(obj,this,24.2f,15);
Commands->Start_Timer(obj,this,24.8f,16);
Commands->Start_Timer(obj,this,25.4f,17);
Commands->Start_Timer(obj,this,26.6f,18);
Commands->Start_Timer(obj,this,27.2f,19);
Commands->Start_Timer(obj,this,28.2f,20);
Commands->Start_Timer(obj,this,28.8f,21);
Commands->Start_Timer(obj,this,29.6f,22);
Commands->Start_Timer(obj,this,30.2f,23);
Commands->Start_Timer(obj,this,31.2f,24);
Commands->Start_Timer(obj,this,42.2f,25);
}
void reb_GDI_ion_storm::Timer_Expired(GameObject *obj, int number) {
char ionstormmsg[128];
if(number == 1){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0097i1evag_snd.wav");
}
if(number == 2){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0076i1evag_snd.wav");
}
if(number == 3){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0098i1evag_snd.wav");
}
/////////////////////////////////////
// numer count down 5,4,3,2,1
/////////////////////////////////////
if(number == 4){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0082i1evag_snd.wav");
sprintf(ionstormmsg,"msg 5");
Console_Input(ionstormmsg);
}
if(number == 5){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0083i1evag_snd.wav");
sprintf(ionstormmsg,"msg 4");
Console_Input(ionstormmsg);
}
if(number == 6){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0084i1evag_snd.wav");
sprintf(ionstormmsg,"msg 3");
Console_Input(ionstormmsg);
}
if(number == 7){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0085i1evag_snd.wav");
sprintf(ionstormmsg,"msg 2");
Console_Input(ionstormmsg);
}
if(number == 8){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0086i1evag_snd.wav");
sprintf(ionstormmsg,"msg 1");
Console_Input(ionstormmsg);
}
////////////////////////////////
// actual storm starts here
////////////////////////////////
if(number == 9){
Vector3 position;
position = Commands->Get_Position(Find_War_Factory(1));
position.Y += 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 10){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(1));
position.Y -= 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 11){
Vector3 position;
position = Commands->Get_Position(Find_Soldier_Factory(1));
position.X += 15.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 12){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(1));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float refhealth = Commands->Get_Health(Find_Refinery(1));
Commands->Set_Health((Find_Refinery(1)),(refhealth/1.3f));
}
if(number == 13){
Vector3 position;
position = Commands->Get_Position(Find_War_Factory(1));
position.Y -= 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 14){
Vector3 position;
position = Commands->Get_Position(Find_Soldier_Factory(1));
position.Y -= 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 15){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(1));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float agthealth = Commands->Get_Health(Find_Base_Defense(1));
Commands->Set_Health((Find_Base_Defense(1)),(agthealth/1.4f));
}
if(number == 16){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(1));
position.X += 10.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 17){
Vector3 position;
position = Commands->Get_Position(Find_Soldier_Factory(1));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float barhealth = Commands->Get_Health(Find_Soldier_Factory(1));
Commands->Set_Health((Find_Soldier_Factory(1)),(barhealth/1.35f));
}
if(number == 18){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(1));
position.Y -= 10.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 19){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(1));
position.X += 5.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 20){
Vector3 position;
position = Commands->Get_Position(Find_War_Factory(1));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float wepshealth = Commands->Get_Health(Find_War_Factory(1));
Commands->Set_Health((Find_War_Factory(1)),(wepshealth/1.4f));
}
if(number == 21){
Vector3 position;
position = Commands->Get_Position(Find_War_Factory(1));
position.X += 10.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 22){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(1));
position.Y += 15.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 23){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(1));
position.X += 5.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 24){
Vector3 position;
position = Commands->Get_Position(Find_Power_Plant(1));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float pphealth = Commands->Get_Health(Find_Power_Plant(1));
Commands->Set_Health((Find_Power_Plant(1)),(pphealth/1.3f));
//////////////////////////////////
/// turn the weather off
///////////////////////////////////
Commands->Set_Fog_Range (0.5f,350.0f,12.0f);
Commands->Set_Rain(0.0f,10.0f,true);
Commands->Set_Wind(0.0f,0.0f,0.0f,3.5f);
}
if(number == 25){
Commands->Set_Fog_Enable(0);
sprintf(ionstormmsg,"msg The ion-storm has sub-sided.");
Console_Input(ionstormmsg);
}
}
//Nod version
void reb_Nod_ion_storm::Created(GameObject *obj) {
// And he said "let there be weather!"
Commands->Set_Rain(10.0f,3.5f,true);
Commands->Set_Fog_Enable(1);
Commands->Set_Fog_Range (0.5,55,3.5f);
Commands->Set_Wind(0.7f,2.0f,1.0f,3.5f);
char ionstormmsg[128];
char ionstormmsg2[128];
Commands->Create_2D_WAV_Sound("m00evag_dsgn0069i1evag_snd.wav");
sprintf(ionstormmsg,"msg Warning!!!");
Console_Input(ionstormmsg);
sprintf(ionstormmsg2,"msg Ion Storm approaching...");
Console_Input(ionstormmsg2);
Commands->Start_Timer(obj,this,3.0f,1);
Commands->Start_Timer(obj,this,4.0f,2);
Commands->Start_Timer(obj,this,4.7f,3);
Commands->Start_Timer(obj,this,14.7f,4);
Commands->Start_Timer(obj,this,15.7f,5);
Commands->Start_Timer(obj,this,16.7f,6);
Commands->Start_Timer(obj,this,17.7f,7);
Commands->Start_Timer(obj,this,18.7f,8);
Commands->Start_Timer(obj,this,19.7f,9);
Commands->Start_Timer(obj,this,20.4f,10);
Commands->Start_Timer(obj,this,21.4f,11);
Commands->Start_Timer(obj,this,22.0f,12);
Commands->Start_Timer(obj,this,23.0f,13);
Commands->Start_Timer(obj,this,23.6f,14);
Commands->Start_Timer(obj,this,24.2f,15);
Commands->Start_Timer(obj,this,24.8f,16);
Commands->Start_Timer(obj,this,25.4f,17);
Commands->Start_Timer(obj,this,26.6f,18);
Commands->Start_Timer(obj,this,27.2f,19);
Commands->Start_Timer(obj,this,28.2f,20);
Commands->Start_Timer(obj,this,28.8f,21);
Commands->Start_Timer(obj,this,29.6f,22);
Commands->Start_Timer(obj,this,30.2f,23);
Commands->Start_Timer(obj,this,31.2f,24);
Commands->Start_Timer(obj,this,42.2f,25);
}
void reb_Nod_ion_storm::Timer_Expired(GameObject *obj, int number) {
char ionstormmsg[128];
if(number == 1){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0097i1evag_snd.wav");
}
if(number == 2){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0076i1evag_snd.wav");
}
if(number == 3){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0098i1evag_snd.wav");
}
/////////////////////////////////////
// numer count down 5,4,3,2,1
/////////////////////////////////////
if(number == 4){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0082i1evag_snd.wav");
sprintf(ionstormmsg,"msg 5");
Console_Input(ionstormmsg);
}
if(number == 5){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0083i1evag_snd.wav");
sprintf(ionstormmsg,"msg 4");
Console_Input(ionstormmsg);
}
if(number == 6){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0084i1evag_snd.wav");
sprintf(ionstormmsg,"msg 3");
Console_Input(ionstormmsg);
}
if(number == 7){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0085i1evag_snd.wav");
sprintf(ionstormmsg,"msg 2");
Console_Input(ionstormmsg);
}
if(number == 8){
Commands->Create_2D_WAV_Sound("m00evag_dsgn0086i1evag_snd.wav");
sprintf(ionstormmsg,"msg 1");
Console_Input(ionstormmsg);
}
////////////////////////////////
// actual storm starts here
////////////////////////////////
if(number == 9){
Vector3 position;
position = Commands->Get_Position(Find_Airstrip(0));
position.Y += 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 10){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(0));
position.Y -= 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 11){
Vector3 position;
position = Commands->Get_Position(Find_Soldier_Factory(0));
position.X += 15.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 12){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(0));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float refhealth = Commands->Get_Health(Find_Refinery(0));
Commands->Set_Health((Find_Refinery(0)),(refhealth/1.3f));
}
if(number == 13){
Vector3 position;
position = Commands->Get_Position(Find_Airstrip(0));
position.Y -= 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 14){
Vector3 position;
position = Commands->Get_Position(Find_Soldier_Factory(0));
position.Y -= 20.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 15){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(0));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float obhealth = Commands->Get_Health(Find_Base_Defense(0));
Commands->Set_Health((Find_Base_Defense(0)),(obhealth/1.4f));
}
if(number == 16){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(0));
position.X += 10.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 17){
Vector3 position;
position = Commands->Get_Position(Find_Soldier_Factory(0));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float handhealth = Commands->Get_Health(Find_Soldier_Factory(0));
Commands->Set_Health((Find_Soldier_Factory(0)),(handhealth/1.35f));
}
if(number == 18){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(0));
position.Y -= 10.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 19){
Vector3 position;
position = Commands->Get_Position(Find_Refinery(0));
position.X += 5.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 20){
Vector3 position;
position = Commands->Get_Position(Find_Airstrip(0));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float striphealth = Commands->Get_Health(Find_Airstrip(0));
Commands->Set_Health((Find_Airstrip(0)),(striphealth/1.4f));
}
if(number == 21){
Vector3 position;
position = Commands->Get_Position(Find_Airstrip(0));
position.X += 10.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 22){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(0));
position.Y += 15.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 23){
Vector3 position;
position = Commands->Get_Position(Find_Base_Defense(0));
position.X += 5.0f;
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
}
if(number == 24){
Vector3 position;
position = Commands->Get_Position(Find_Power_Plant(0));
Commands->Create_2D_WAV_Sound("ion_fire.wav");
Commands->Create_Explosion("Explosion_Mine_Remote_01",position,obj);
Commands->Create_Object("Beacon_Ion_Cannon_Anim_Post",position);
float pphealth = Commands->Get_Health(Find_Power_Plant(0));
Commands->Set_Health((Find_Power_Plant(0)),(pphealth/1.3f));
//////////////////////////////////
/// turn the weather off
///////////////////////////////////
Commands->Set_Fog_Range (0.5f,350.0f,12.0f);
Commands->Set_Rain(0.0f,10.0f,true);
Commands->Set_Wind(0.0f,0.0f,0.0f,3.5f);
}
if(number == 25){
Commands->Set_Fog_Enable(0);
sprintf(ionstormmsg,"msg The ion-storm has sub-sided.");
Console_Input(ionstormmsg);
}
}
ScriptRegistrant<reb_GDI_ion_storm> reb_GDI_ion_storm_Registrant("reb_GDI_ion_storm","");
ScriptRegistrant<reb_Nod_ion_storm> reb_Nod_ion_storm_Registrant("reb_Nod_ion_storm","");
class reb_GDI_ion_storm : public ScriptImpClass {
void Created(GameObject *obj);
void Timer_Expired(GameObject *obj,int number);
};
class reb_Nod_ion_storm : public ScriptImpClass {
void Created(GameObject *obj);
void Timer_Expired(GameObject *obj,int number);
};
For testing I called these functions by a chat hook. Obviously you're going to want to call the functions some other way, perhaps a crate, a random weather effect or some other way.
But here is my chat hook for it anyway.
class GDIionstormChatCommand : public ChatCommandClass {
void Triggered(int ID,const TokenClass &Text,int ChatType) {
GameObject *obj = Get_GameObj(ID);
Commands->Attach_Script(obj,"reb_GDI_ion_storm","");
}
};
ChatCommandRegistrant<GDIionstormChatCommand> GDIionstormChatCommandReg("!iong",CHATTYPE_TEAM,0,GAMEMODE_AOW);
class NodionstormChatCommand : public ChatCommandClass {
void Triggered(int ID,const TokenClass &Text,int ChatType) {
GameObject *obj = Get_GameObj(ID);
Commands->Attach_Script(obj,"reb_Nod_ion_storm","");
}
};
ChatCommandRegistrant<NodionstormChatCommand> NodionstormChatCommandReg("!ionn",CHATTYPE_TEAM,0,GAMEMODE_AOW);
|
|
|
|
|
|
|
|
|
Re: ion storm effect function [message #300966 is a reply to message #300917] |
Tue, 04 December 2007 11:28   |
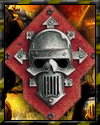 |
IronWarrior
Messages: 2460 Registered: November 2004 Location: England UK
Karma: 0
|
General (2 Stars) |
|
|
Jerad Gray wrote on Tue, 04 December 2007 09:06 | Lol, you even have it set up to hit the buildings, just like in TS. Nice. Oh, I guess you say that in the video, I was just reading through the code.
|
Smart arse. ^^
Ah Reborn, I know what you going though, but when you though it, I do hope you get time to make the uber mod you doing, I've looking forward to it.
|
|
|
|
|
|
Re: ion storm effect function [message #301058 is a reply to message #301055] |
Tue, 04 December 2007 16:14   |
 |
havoc9826
Messages: 562 Registered: April 2006 Location: California, USA
Karma: 0
|
Colonel |
|
|
Renardin6 wrote on Tue, 04 December 2007 15:06 | awesome. I want it. Any video preview?
|
Now if only the fog could be yellow, the ion effects replaced with lightning bolts, and put on MutationRedux... Also, if this actually gets into C&C Reborn as a crate or a feature of specific maps, remember to make it disable radar and hover/flying vehicles.
[Updated on: Tue, 04 December 2007 16:25] Report message to a moderator
|
|
|
|
|
|
Re: ion storm effect function [message #307256 is a reply to message #300900] |
Tue, 01 January 2008 22:18   |
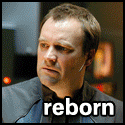 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
If a player is near the explosion then yes, they will recieve some damage. The explosions do not just happen at the same position as the buildings, I just use the building positions so that it can be used dynamically for any map without having to hard code it for all them.
I'm not sure is there is a bolt like animation that lasts for a second :-/
|
|
|
|
Re: ion storm effect function [message #315718 is a reply to message #300900] |
Wed, 06 February 2008 03:56   |
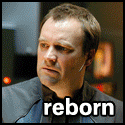 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
Ah, if your attaching the script tot he player object and the player dies then the script would get destroyed. But I guess setting the weather functions means they would stay on...
Try attaching the script to an invisible object instead of the player obj.
|
|
|
Re: ion storm effect function [message #315792 is a reply to message #300900] |
Wed, 06 February 2008 11:26   |
Genesis2001
Messages: 1397 Registered: August 2006
Karma: 0
|
General (1 Star) |
|
|
When the player picks up the crate, use Commands->Get_Postion(obj) on the player to get the player's position. Then Commands->Create_Object("invisible_object", <player's position>) then attach the script to that object. ^,^
~Zack
|
|
|
Re: ion storm effect function [message #315796 is a reply to message #300900] |
Wed, 06 February 2008 11:37   |
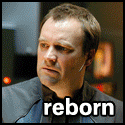 |
reborn
Messages: 3231 Registered: September 2004 Location: uk - london
Karma: 0
|
General (3 Stars) |
|
|
The invisible obj can be created anywhere on the map really, doesn't need to be at the players position. You could hard code the spawn location at 0,0,0 if you wanted to...
The weather effects happens globally on the map, but the ion blasts happen around the base building's, not to the player/obj the script is attached to. It wouldn't be too hard to make it happen around random players though aswell...
|
|
|
|
|
|
|
Goto Forum:
Current Time: Tue Jul 29 21:32:23 MST 2025
Total time taken to generate the page: 0.01491 seconds
|